Hi, If you are a Laravel learner then you may have used routes in laravel rather than creating them one by one for each crud operation we can do this by a single line of code.
See the code snippet below.
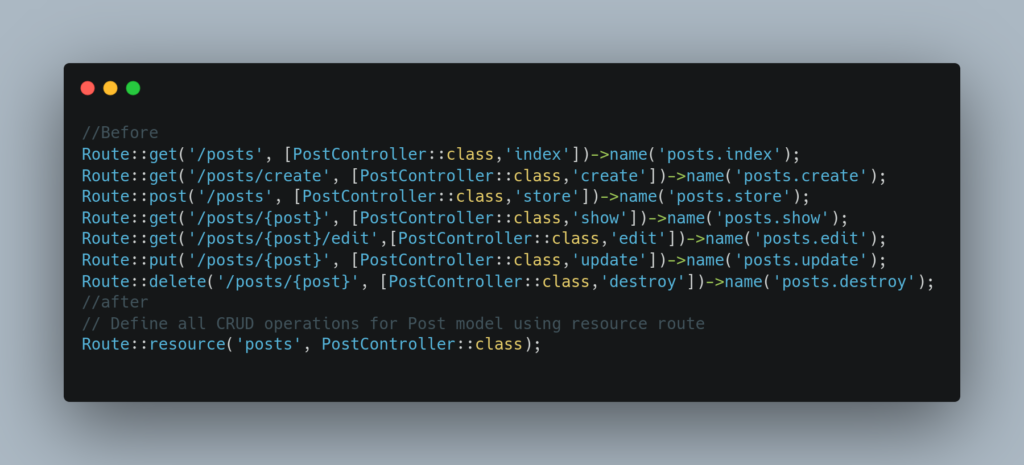
Why Resource Routes
When building a web application in Laravel, you need to define routes that map to the various actions that your application can perform. For simple applications, you might be able to get away with defining individual routes for each action. However, for more complex applications, this can quickly become unwieldy.
That’s where resource routes come in. Resource routes are a way of defining a set of routes that map to the various CRUD (Create, Read, Update, and Delete) operations for a resourceful controller. By using resource routes, you can quickly define all of the necessary routes for your application in a single line of code.
Defining Resource Routes
Resource routes in Laravel are defined using the Route::resource
method. Here’s an example:
Route::resource('posts', PostController::class);
In this example, we define a set of resource routes for the PostController
. Laravel will automatically generate routes for each of the CRUD operations, as well as a few additional routes:
Method | URI | Action | Route Name |
---|---|---|---|
GET | /posts | index | posts.index |
GET | /posts/create | create | posts.create |
POST | /posts | store | posts.store |
GET | /posts/{post} | show | posts.show |
GET | /posts/{post}/edit | edit | posts.edit |
PUT/PATCH | /posts/{post} | update | posts.update |
DELETE | /posts/{post} | destroy | posts.destroy |
The Route::resource
the method generates these routes based on RESTful conventions. Here’s what each route does:
GET /posts
(posts
.index): Lists all postsGET /posts/create
(posts
.create): Displays a form for creating a new postPOST /posts
(posts
.store): Creates a new postGET /posts/{<code><code><code><code>post
} (posts
.show): Displays a specific postGET /posts/{<code><code><code><code>post
}/edit (posts
.edit): Displays a form for editing a postPUT/PATCH /posts/{post}
(posts
.update): Updates a specific postDELETE /posts/{<code><code><code>post
} (posts
.destroy): Deletes a specific post
Customizing Resource Routes
While the default resource routes are convenient, you may want to customize them to better fit your application’s needs. Laravel provides several options for customizing resource routes:
Specifying Which Routes to Generate
By default, Route::resource
generates all of the resource routes. However, you can use the only
and except
options to specify which routes to generate. For example, if you only want to generate the index
, show
, and destroy
routes, you can do this:
Route::resource('posts', PostController::class)->only(['index', 'show', 'destroy']);
Similarly, if you want to generate all of the routes except for the create
and edit
routes, you can do this:
Route::resource('posts', PostController::class)->except(['create', 'edit']);
Naming Resource Routes
The next thing we need to discuss regarding resource routes is naming them. By default, Laravel generates resource routes with names based on the resource name and HTTP verb. For example, a GET request to /posts
generates a route named posts.index
, while a POST request to /<code>posts
generates a route named <code>posts
.store.
However, you may want to customize these names to fit your application’s naming conventions. To do so, you can pass a second argument to the Route::resource
method with an array of options. One of these options is, names
which allows you to customize the names of the generated routes.
Here’s an example of how to customize the names of a resource:
Route::resource('posts', 'PostsController', [
'names' => [
'index' => 'posts.list',
'show' => 'posts.view',
'create' => 'posts.add',
'edit' => 'posts.edit',
'update' => 'posts.update',
'store' => 'posts.save',
'destroy' => 'posts.delete',
]
]);
In this example, we’ve customized the names of all the resource routes for the <code>posts
resource. For example, the posts.index
route is now named <code>posts
.list, and the <code>posts
.show route is named <code>posts
.view. You can customize the names of as many or as few routes as you like.
Customizing the names of your resource routes can make your code more readable and easier to understand, especially if you have a lot of routes in your application. It also makes it easier to generate URLs for your resource routes using the route
helper function.
In conclusion, resource routes in Laravel are a powerful and convenient way to define all the CRUD routes for a given resource in a single line of code. They provide a standardized way of naming and organizing your routes, making it easier to maintain and understand your application’s codebase. By default, resource routes use a set of conventions for naming and generating routes, but you can customize these names to fit your application’s needs using the names
option.
Beginner: Service Classes In Laravel
Conclusion
In this post, we’ve covered the basics of using resource routes in Laravel to define CRUD routes for your application’s resources in a single line of code. We’ve discussed how resource routes follow a standardized naming convention, making it easy to organize and maintain your application’s routes. I hope this will help.
2 thoughts on “A Beginner’s Guide to Resource Routes in Laravel”