Hello everyone, after a bit long time I thought that let’s do something now. Coz I stopped learning new things a few weeks ago. Then I started working on a core PHP project that I have in my organization. But the issue was the structure was too linear. I decided to move to OOPs. Because it feels more cool and smart. But as you know that we shouldn’t forget the DRY way ever. If we can write a few lines of code that can help us not to repeat ourselves again and again, then I would say that we should spend some time on this kind of thing.
So, I wrote a few classes for DB operations like -> Insert, update, create, and read. Basically for crud operations. Not advanced relationship etc. So, I wrote those classes and I said I wrote a small and simple query builder. I took help from chatGpt as we all can do this. But it was an amazing experience for me. I hope this will help you to build your first composer package in PHP.
How It Started
After doing this in my organization, I came home and thought that what’s there to work on this weekend. Well, nothing was there. I thought that let’s build this query builder as a small and simple library in PHP. Before this, I didn’t have any experience in publishing and building a package from scratch. So, I watched a few videos on Youtube that how can I start, and then after getting some knowledge about it I started building the package.
Then I started doing the initial stuff. And I built querybuilder so, I’ll be taking the example of this package.
Let’s understand what you need to build any package.
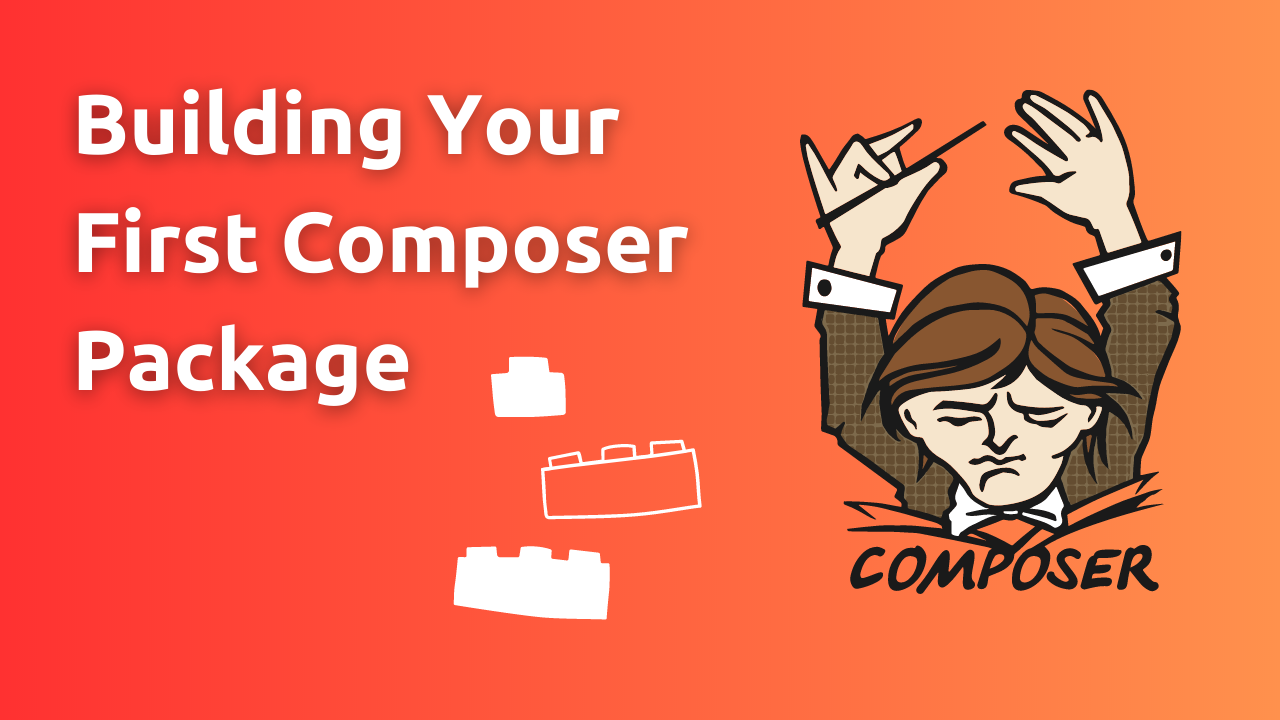
Requirements
- Basic understanding of PHP programming.
- Familiarity with the command line.
- Composer installed on your development machine.
Later I did the project setup, And I should say this that I changed the structure more than 5 times.
Project Setup
querybuilder/
|- src/
| |- Greeting.php
|- composer.json
Define Your Class
In the src/
directory, create a PHP class that you want to share as a package. For example, let’s create a simple class to greet users:
<?php
namespace robinksp\querybuilder;
class Greeting
{
public function greet($name)
{
return "Hello, $name!";
}
}
Create composer.json
In the root of your package directory, create a composer.json
file to define your package’s metadata, dependencies, and autoloading. Here’s a basic example:
{
"name": "robinksp/querybuilder",
"description": "A simple PHP querybuilder for your php projects.",
"type": "library",
"license": "MIT",
"require": {
"php": "^7.4"
},
"autoload": {
"psr-4": {
"robinksp\\querybuilder\\": "src/"
}
}
}
How to Test?
Well, I tested the package locally right after building this. Because as I said initially I didn’t know how I have to build this after spending a lot of time I understood the flow a little bit and I created another directory called crud and in this directory, I have added the simple form. In I was taking the values and now the challenge was how can I use the local package in it.
Then to use the local package I created a composer.json
file in this folder and added the following lines.
{
"repositories": [
{
"type": "path",
"url": "C:/wamp64/www/Packages/querybuilder",
"options": {
"symlink": false
}
}
],
"require": {
"robinksp/querybuilder": "^1.0"
}
}
And to test it is working or not I did a test code as following :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form method="post">
<input type="text" name="first_name" value="Robin" />
<input type="text" name="last_name" value="Kashap" />
<button type="submit" name="submit"> Submit </button>
</form>
</body>
</html>
<?php
ini_set('display_errors',1);
require 'vendor/autoload.php';
use robinksp\querybuilder\Query;
if(isset($_POST['submit'])){
unset($_POST['submit']);
try {
$qb = new Query();
$qb->table('users')->insert($_POST);
echo 'Inserted';
} catch (\Throwable $th) {
echo 'Failed Because: '. $th->getMessage();
}
}
And I was happy coz it was working.
HAHAHA. But this was an amazing experience.
Publish on GitHub
So, you have to publish on GitHub to make this publicly accessible.
Create a new repository on GitHub for your package. Push your code, including the composer.json
file, to the repository.
Publish On Packagist
Go to https://packagist.org/ and create an account if you haven’t already. Click “Submit” in the top-right corner and provide the URL of your GitHub repository. Your package is now published on Packagist.
Install Your Package
Now, you can install your package in any PHP project using Composer:
composer require yourvendor/your-package
Related: Understanding Dependency Injection
Conclusion
Congratulations! You’ve successfully built and published your first Composer package. Your code is now accessible to developers worldwide, and they can easily include it in their projects using Composer. Building and sharing packages not only help others but also promotes code reuse and collaboration in the PHP community.
Remember, building packages is an ongoing process. As you enhance your package or fix issues, don’t forget to tag new releases on GitHub and update the composer.json
accordingly. Happy coding and sharing!
1 thought on “Building Your First Composer Package”