Hi, It’s very easy to add a Github login to your laravel application. Let’s understand how we can do this. So, I’m going to practical example now.
Create Github OAuth App
Now, you have to go to your GitHub account in your account go to Settings>Developer settings>OAuth Apps, and create your app there.
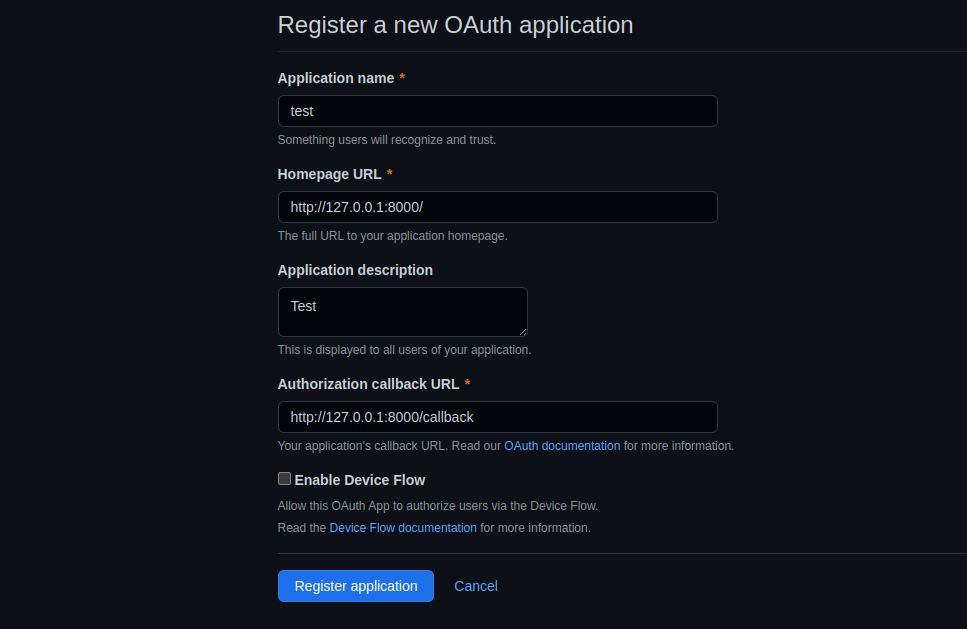
now you have created your app. You will get the secret key and Client id. Come back to your laravel application and do the next steps.
Install A Socialite Package
I’m assuming that you have already installed the Socialite package so that we can implement the GitHub Login in our application. if you are using Breeze or any other authentication package then you have to manage it accordingly, I think you just have to change the paths not anything extra to implement the social GitHub login.
let’s install Socialite:
composer require laravel/socialite
Register Socialite
In Config>app.php register socialite.
'providers' => [
/*
* Package Service Providers...
*/
Laravel\Socialite\SocialiteServiceProvider::class,
],
'aliases' => Facade::defaultAliases()->merge([
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
])->toArray(),
Add Keys Secret And Client ID
Now, you have to add the keys in config>services.php you can define keys in `.env` or user directly.
'github' => [
'client_id' => GITHUB_CLIENT_ID,
'client_secret' => GITHUB_CLIENT_SECRET,
'redirect' => 'http://127.0.0.1:8000/callback',
],
Create View
I have created a login button in welcome.blade.php
you can do according to your needs.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Github Login</title>
</head>
<body>
<div style="display:flex;justify-content:center;align-items:center;min-height:100vh;background:rgb(241, 187, 187);">
<form action="{{route('login.github')}}" >
@csrf
<button style="background:rgb(28, 28, 28);padding:10px;color:white;">Login with Github</button>
</form>
</div>
</body>
</html>
This will look like this.
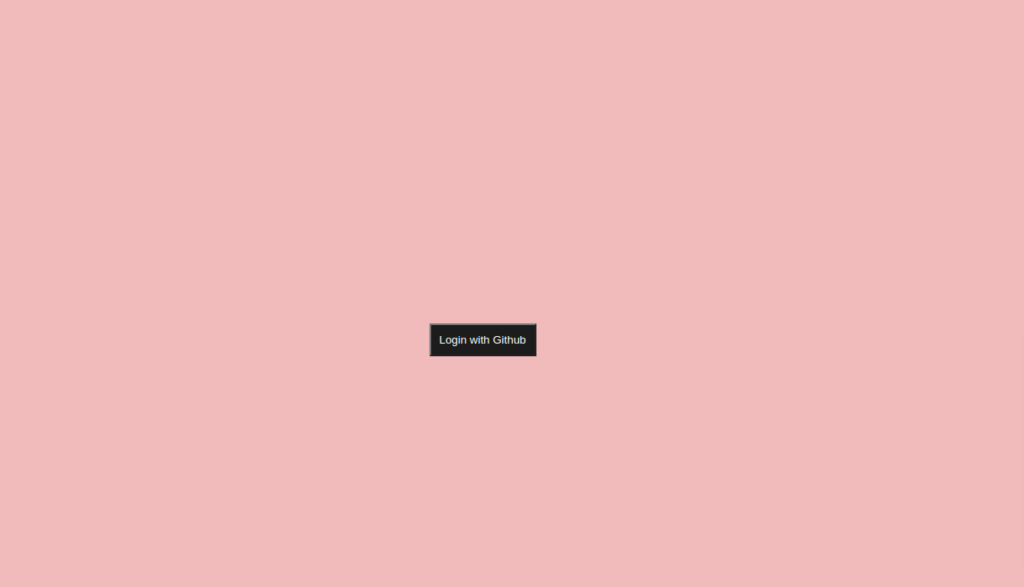
After this, I created a controller.
Create a Controller To Handle the Login
php artisan make:controller LoginController
if you have, you don’t need to create just implement the methods in that controller with `routes`.
LoginController
Will look like this:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Laravel\Socialite\Facades\Socialite;
class LoginController extends Controller
{
public function index()
{
return view('welcome');
}
public function redirectToGithub()
{
return Socialite::driver('github')->redirect();
}
public function callback()
{
$user = Socialite::driver('github')->user();
dd($user); //do whatever with user data
}
}
Create Routes
Now we will create routes.
Route::get('/', function () {
return view('welcome');
});
Route::get('/callback', [LoginController::class , 'callback']);
Route::get('/login/github', [LoginController::class , 'redirectToGithub'])->name('login.github')->middleware('guest');
Read Also: Resource Route In Laravel explained
Now you are ready to go. Thanks for reading I hope it will help you.
is the same process if i create a Github app instead Oauth App ?
i haven’t tried