Hey there, fellow devs!
Today, we are going to learn (axios) how to send Axios requests to a Laravel backend from ReactJS. It’s one of those things that sounds straightforward but can get a bit tricky if you don’t know the ropes. So, let’s dive in and ensure you’re handling those axios requests like a pro.
Setting Up Axios
First things first, let’s make sure you have Axios installed. If you haven’t already, run this in your project directory
npm install axios
Got it? Great! Now, let’s set up a basic Axios instance. This will help us manage our requests more efficiently.
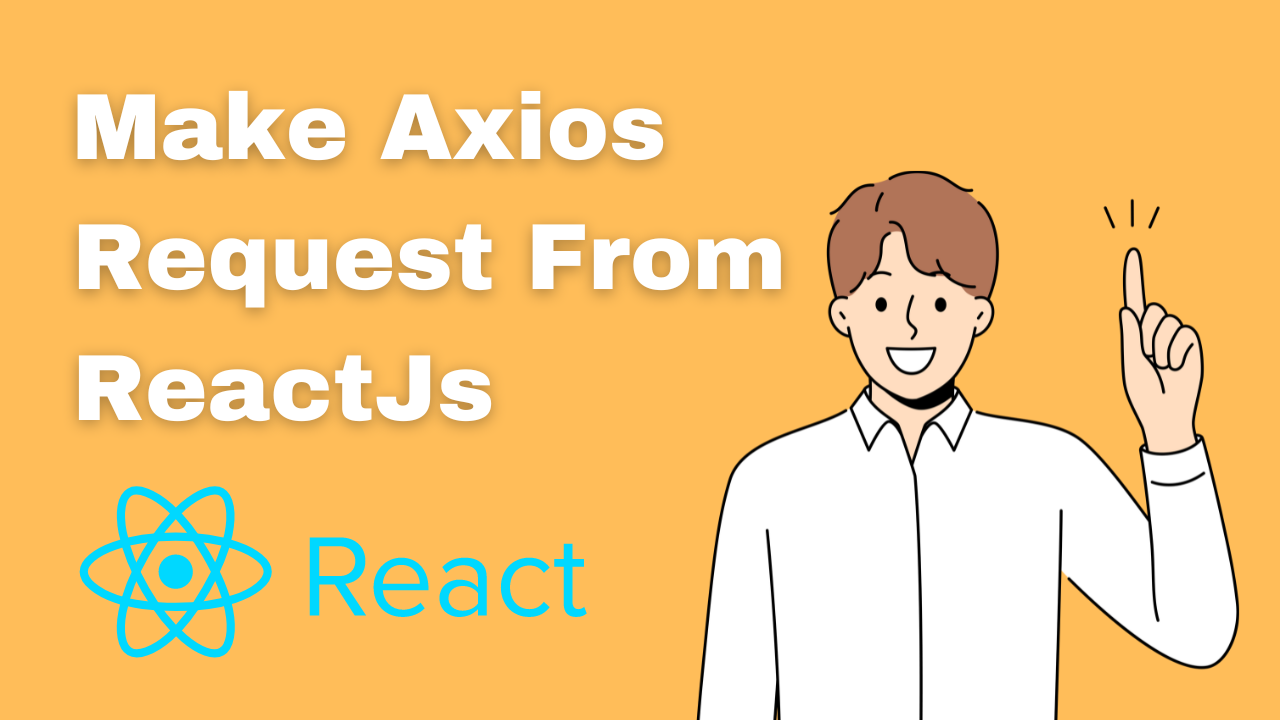
import axios from 'axios';
const api = axios.create({
baseURL: 'http://your-laravel-backend-url/api',
headers: {
'Content-Type': 'application/json',
},
});
export default api;
Making The First Request
Let’s say you want to fetch some user data from your Laravel backend. Here’s how you can do it:
import React, { useEffect, useState } from 'react';
import api from './api';
const UserList = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchUsers = async () => {
try {
const response = await api.get('/users');
setUsers(response.data);
} catch (error) {
console.error('Error fetching users:', error);
}
};
fetchUsers();
}, []);
return (
<div>
<h1>User List</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
};
export default UserList;
Handling Authentication In Axios
Most of the time, you’ll need to handle authentication. Here’s how you can include a token in your Axios requests most of the time, you’ll need to handle authentication. Here’s how you can include a token in your Axios requests.
import axios from 'axios';
const api = axios.create({
baseURL: 'http://your-laravel-backend-url/api',
headers: {
'Content-Type': 'application/json',
},
});
//adding a request interceptor
api.interceptors.request.use(config => {
const token = document.querySelector('meta[name="csrf-token"]').getAttribute('content');
if (token) {
config.headers['X-CSRF-TOKEN'] = token;
}
const authToken = localStorage.getItem('token');
if (authToken) {
//if you have auth token kind of thing to send requests.
config.headers.Authorization = `Bearer ${authToken}`;
}
return config;
}, error => {
return Promise.reject(error);
});
export default api;
Posting Data Through Axios
Need to send some data to your backend? Here’s a simple example of how to handle that using axios:
const createUser = async (userData) => {
try {
const response = await api.post('/users', userData);
console.log('User created:', response.data);
} catch (error) {
console.error('Error creating user:', error);
}
};
const handleSubmit = (event) => {
event.preventDefault();
const userData = {
name: 'John Doe',
email: 'john@example.com',
password: 'password123',
};
createUser(userData);
};
Error Handling
Error handling is crucial. You don’t want your users staring at a blank screen when something goes wrong. Here’s a better way to handle errors
const fetchUsers = async () => {
try {
const response = await api.get('/users');
setUsers(response.data);
} catch (error) {
if (error.response) {
console.error('Server responded with a status other than 200 range:', error.response);
} else if (error.request) {
console.error('No response was received:', error.request);
} else {
console.error('Error setting up the request:', error.message);
}
}
};
Recommended: I may will add some resources to this page you can bookmark if you want.
Conclusion
There you have it, folks! This should get you started with making Axios(Read documentation as well) requests to your Laravel backend like a pro. Remember, the key is to stay organized and handle your requests and errors properly. Happy coding, and may your APIs always respond quickly!
Feel free to ask any questions or share your experiences in the comments. Let’s learn together!