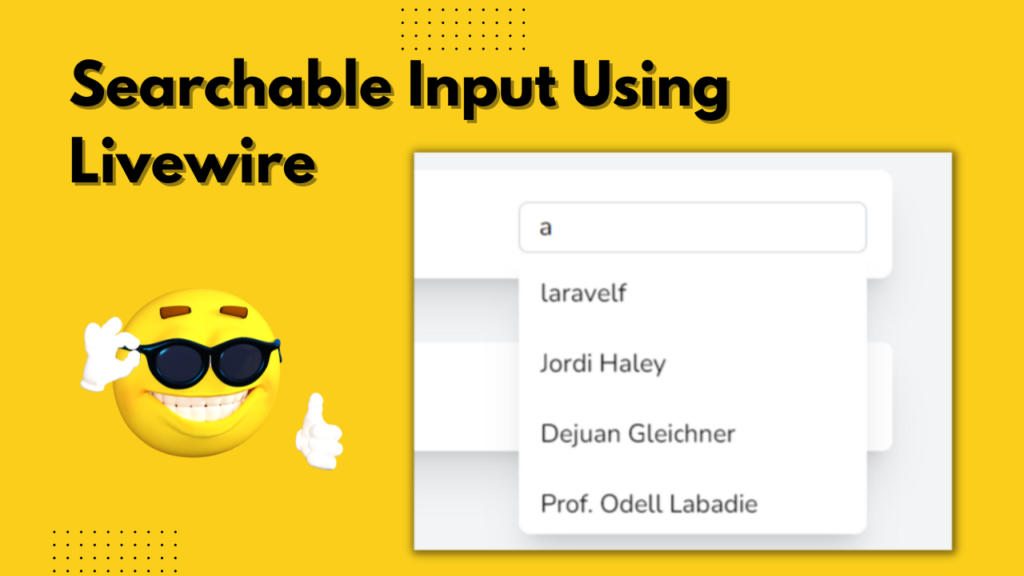
Hi, Gurpreet this side with some new stuff today. Let me tell you, what we will cover in this article. We will create a searchable input in which we can search records using livewire and with that we can show the records in dropdown. What I am gonna use to make this stuff. I will be using Tailwind to design stuff if you are familiar with any other framework of css you can simply use that to create the same design.
But the main thing about logic.
Project Description
I am working on a Task management web application and now I have added some tasks into the task box. I want to share these tasks with my team members and also want to assign them. For that what I have to do, We have to invite them or we can just search their names and put them in.
Prerequisites
Install Laravel and Livewire
I assume that you are a learner and you know that how to install Laravel. It depends on you what you want to use with Livewire. Because here you have to options first is Jetstream and second is Breeze. I haven’t used Livewire with breeze yet but the functionality we are going to do, This all will get done by Livewire itself.
Now, I assume that you have installed all packages whatever you need to run with Livewire. Now main focus on Livewire.
Connect Database and Seed
I hope you might have understood about the requirement. And now you should have some users in your database for that you can easily seed the Database seeder because with that you can create multiple record as much as you want.
\App\Models\User::factory(10)->create();
run migration and finally we are ready to go.
After seeding the database you will have some records. Now we are ready to go.
Create Livewire Input Component
Now, we will create a component. If you are not so much of familiar with Livewire you can just practice Insert in Livewire. Some points I have deeply shared in this article. How to insert data into database using Livewire
php artisan make:livewire invite_members
You will have a class and component of livewire. Now let’s see what we gonna do in the component. Why I made componen?
You can use it anywhere in any page.
invite-members.blade.php
in under livewire directory in view.
Livewire Input Component Structure
First of all, I have used a in-built Jetstream component to create this input field using Tailwind css and If you want to do the same thing without component then you can also draw the input without component or with any framework.
<div class="col-span-6 sm:col-span-4 relative z-10">
<x-jet-input id="groupname" type="text" class="mt-1 block w-34 h-8 p-3" placeholder="search members..."
wire:model="query" wire:keydown.tab="resetVars" wire:keydown.escape="resetVars" />
<div class="max-h-44 overflow-y-scroll absolute bg-white w-full z-40 rounded-b-lg shadow-xl serachCard " wire:loading>
<ul class="list-outside list-none">
<li
class="p-1.5 border-b-1.5 border-slate-200 cursor-pointer divide-y m-2 hover:rounded-lg">
Searching...</li>
</ul>
</div>
<div class="max-h-44 overflow-y-scroll absolute bg-white w-full z-40 rounded-b-lg shadow-xl serachCard">
@if (!empty($query))
<div class="fixed top-0 right-0 bottom-0 left-0 -z-10" wire:click="resetVars"></div> //to reset the search result
@if (!empty($friends))
@foreach ($friends as $friend)
<ul class="list-outside list-none">
<li
class="p-1.5 border-b-1.5 border-slate-200 cursor-pointer divide-y hover:bg-slate-100 m-2 hover:rounded-lg z-40">
{{$friend['name']}}</li>
</ul>
@endforeach
@else
<ul class="list-outside list-none">
<li
class="p-1.5 border-b-1.5 border-slate-200 cursor-pointer divide-y hover:bg-slate-100 m-2 hover:rounded-lg">
No result found</li>
</ul>
@endif
@endif
</div>
</div>
Note: when we use wire and colon with any attribute that means we are interacting with livewire. Because wire is provided by livewire and it works as a event selector as you might have seen in Javascript or jQuery. In double quotes we target methods or properties from component class.
Searchable Input Component Explanation
wire:model="query"
by using this attribute provided by Livewire we are targeting a property which we will create in our component class. It will send the value of the input to query variable which we will define in class as a public property.wire:keydown.tab="resetVars"
by using this attribute we are saying to livewire that if user will click on tab to this result should be zero and the same goes withwire:keydown.escape
.wire:loading
is provided by livewire so that we can show loading or any pre loader under this when are searching for result on Ajax. It also interacts with sleep() method which you can see in the code below.
Program The Component Class (InviteMembers.php)
I will create all the methods or functions first. Then I’ll explain all the functions how they work. After that we just need to create an input field to handle it all.
//InviteMembers.php
<?php
namespace App\Http\Livewire;
use App\Models\User;
use Livewire\Component;
class InviteMembers extends Component
{
public $query;
public $friends;
public function mount()
{
$this->resetVars(); //updating the result to zero
}
public function resetVars()
{
$this->query = '';
$this->friends = [];
}
public function updated()
{
sleep(1); //to create a difference between search and result of 1 second
$this->friends = User::where('name', 'like', '%' . $this->query . '%')
->get()->toArray();
}
public function render()
{
return view('livewire.invite-members');
}
}
Component Class Explanation
As you have seen that in first we have created the component class. And In input we have used some attributes related to the class and also target some methods.
wire:model="query"
from input and as you can see we have public property called query that we are using to get the value of input box. That’s why we have used query in input wire model. On each keypress that runs a request towards component class.
mount()
is in-built method in Livewire. Which is a part of Livewire lifecycle hooks. Mount runs once when page load and before the component is rendered.
What we are in this mount, we are just setting the result values to null on page load. when user will search through input box only then the user can see the result.
updated()
is also an in-built method in Livewire that works when component data updates. For example, as we have query in target in input box and when this component will get updated then this method will work automatically and push the result of Users.
When we are updating the result with updated lifecycle hook. Then we are putting this result in friends property and you can use public property directly in your component.
Conclusion
It is very easy to make this kind of functionality using livewire and I am happy because for same thing I did write lots of lines of code in jQuery.
well, It really feels stress free when you do use Livewire because here you don’t need to use single of line of code to create functionalities like this.
Thanks ❤